(• Reading Time: 11 minutes •)
The secret to becoming an efficient coder is to build projects.
But don't just jump into any project as a beginner software developer.
Instead, start small.
Build your confidence then expand the scope of the project. I've received a lot of questions on what projects to start with.
So I have one goal: to solve the problem of finding a project once and for all!
Two sections of developer project ideas
I've divided this list of programming project ideas into two parts.
- In part 1, I hand pick and also create new programming projects for beginners. You can jump on them in any programming language of your choice – Python, JavaScript, Java, C++, whatever you favorite language is.
- In part 2, I put together a list of lists. After going through a ton of online programming project lists, I selected only the most useful ones that other developers created and categorized them here.
This list of programming projects covers multiple programming languages from tech leaders committed to helping others.
And the projects span both beginner and beyond beginner levels.
You'll find Python programming project ideas, Javascript projects, Java projects, ideas for mobile app development (android and iOS), and more.
Different formats, too.
You'll find written project as well as youtube tutorials on project ideas if you prefer video over text.
Let's get to it.
Before you you build any programming project
You have to learn the basics.
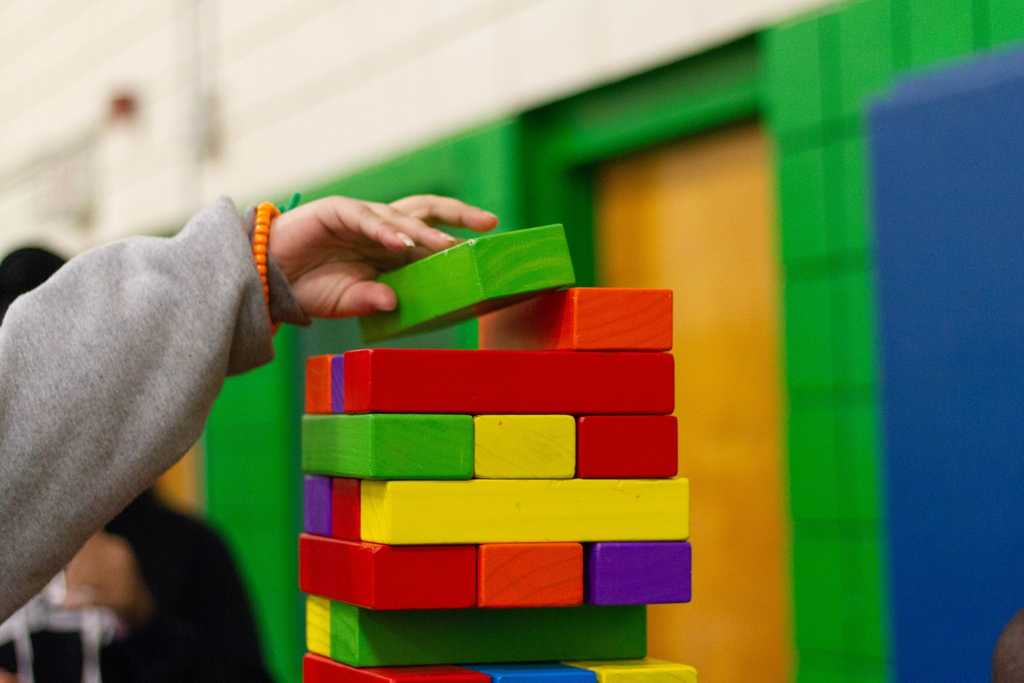
Skip this and you'll have a shaky foundation as a developer. And this will hold you back for a long time.
No matter the level of mastery you hope to get to, you'll need to know how to:
- declare variables
- collect user input
- store information
- repeat an action through loops
- write functions to repeat blocks of code
That's it.
Of course there's more to learn in any programming language you focus on, but these are standard things you need to know and they'll be good enough for you to jump into beginner projects.
There's that tempting feeling that you need to finish lots of Python tutorials before you work on any project.
Don't do it.
Many fall into the trap of learning back to back Python tutorials waiting to feel super ready. Instead, learn the basics first. Next, build some tiny projects. Then return to learning with more tutorials.
Trust me, you can finish 100 Python tutorials and still feel you're not ready to build any projects.
Studying alone is not enough.
You need to build.
How to use programming projects
- First, read through the instructions and make sure you understand what you've read. Try to say what you read in your own words.
- Attempt to solve it on your own without going through the YouTube tutorial or example code on Github. You'll most likely struggle. That's fine. Push yourself. This is the idea of deliberate practice from Behavioral Psychology.
- If you haven't made any progress at all, watch the YouTube tutorial where available then look through the example code. You can also search online to see more example tutorials and Python code for the same problem.
- After going through, go back and try to write the code by yourself without looking at the tutorial. Again, push yourself. This is grit also from Behavioral Psychology. You need it.
- Whatever you do, do not blindly copy out the code in the tutorial, then pat yourself on the back. You may finish quickly but the reality is you haven't learned anything.
- If you feel you're completely stuck after lots of attempts, take a break. When you step away, we know from Neuroscience research that your subconscious will continue the learning. This is because your mind has shifted from focused mode to diffused mode.
- Whenever you solve something, celebrate it! You need the positive reinforcement to build internal references that you did it. In the future when your mind whispers “you can't do it” during a moment of doubt, you'll reply “that's not true, here's proof of what I did in the past so I can do this too”.
- Repeat, repeat, repeat.
You can use this breakdown for any programming project in any language. It works.
Now, lets get into the projects for beginner programmers and junior developers.
Part 1 - Programming projects for beginners and junior developers
- Odd or even
- Mad Libs Game
- Word Count
- Biography info
- What's my acronym?
- Rock, Paper, Scissors
- Guess the number
- Is a palindrome
- Email slicer
- Lyrics generator
Odd or even
Welcome a user then ask them for a number between 1 and 1000.
When the user gives you the number, you check if it's odd or even and then you print a message letting them know.
Mad libs game
Ask the user for an input.
This could be anything such as a name, an adjective, a pronoun or even an action. Once you get the input, you can rearrange it to build up your own story.
Here's a youtube tutorial on mad libs in Python.
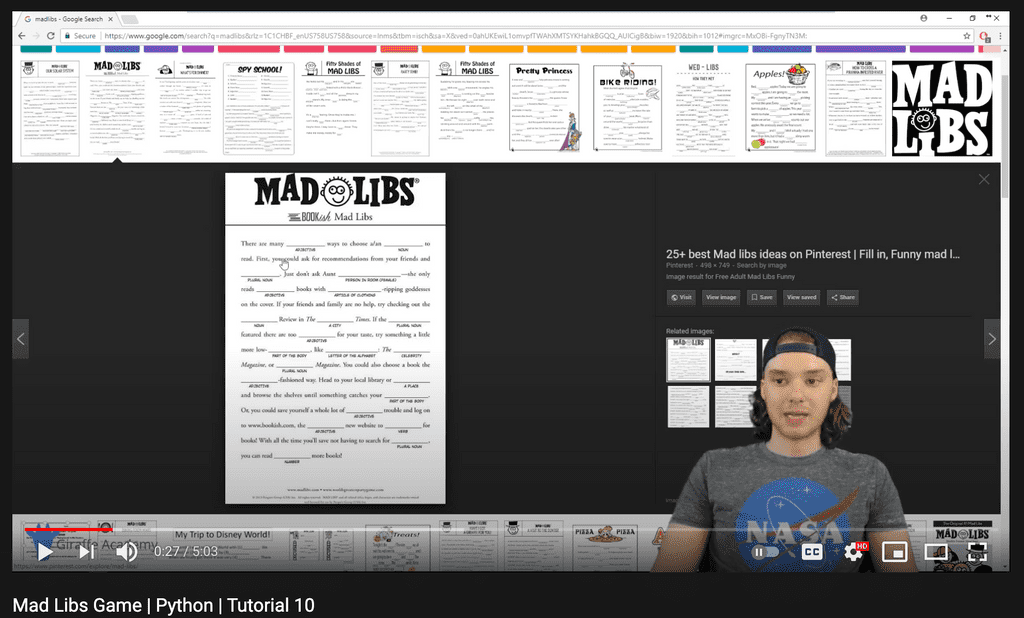
Word count
Ask the user what's on their mind. Then after the user responds, count the number of words in the sentence and print that as an output.
Example:
- Prompt:
what's on your mind today?
- Input:
well, it's just a day for me to be an expert in coding
- Output:
oh nice, you just told me what's on your mind in 13 words!
Biography info
Ask a user for their personal information one by one. Then check that the information they entered is valid. Finally, print a summary of all the information they entered back to them.
Example: What is your name? If the user enters *
you prompt them that the input is wrong. And ask them to enter a valid name. At the end you print a summary like that looks like this:
- Name: John Doe
- Date of birth: Jan 1, 1954
- Address: 24 fifth Ave, NY
- Personal goals: To be the best programmer there ever was.
What's my acronym?
Ask the user to enter the full meaning of an organization or concept and you'll provide the acronym to the user. For example:
-
Input ->
As Soon As Possible
.- Output ->
ASAP
.
- Output ->
-
Input ->
World Health Organization
.- Output ->
WHO
.
- Output ->
-
Input ->
Absent Without Official Leave
.- Output ->
AWOL
.
- Output ->
Rock, Paper, Scissors
This is a popular hand game played between two people. Each player gets to form one of three shapes using their hand:
rock
(a closed fist)paper
(a flat hand)scissors
(a fist with the index finger and middle finger extended, forming a V)
Here's a youtube tutorial on coding up rock-paper-scissors in Python.
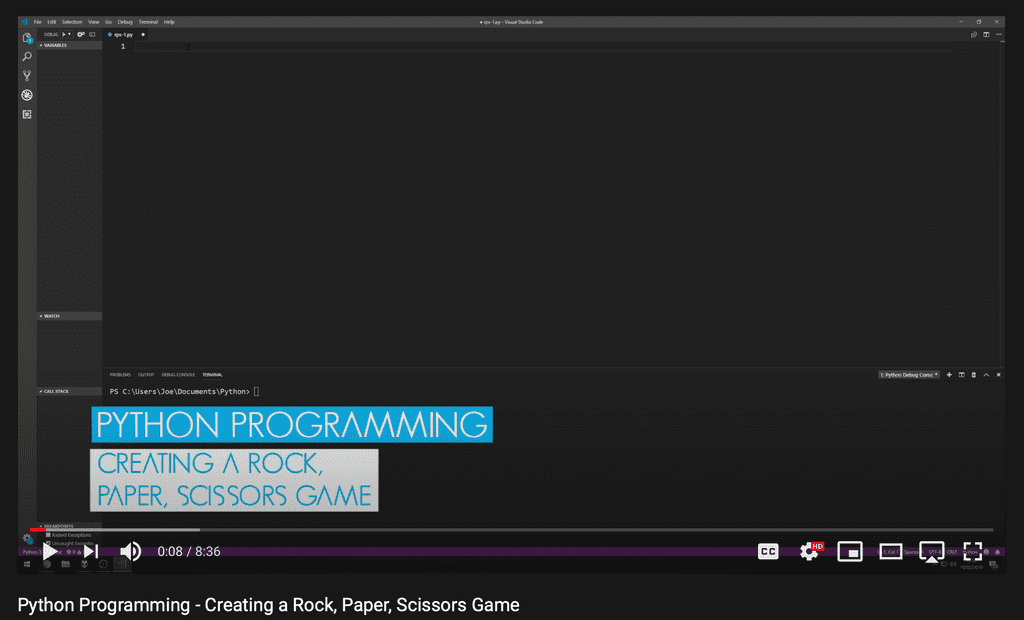
Guess the number
You ask a user to guess a number between 1 and 50.
If they guess outside that range, you prompt with an error encouraging them to choose a number within the proper range.
Whenever they guess the wrong number you ask if they want to keep playing or they'd like to quit.
Finally, when the user eventually guesses the right number you congratulate them and show the number of attempts they had.
Here's a youtube tutorial on coding up guessing a number in Python.
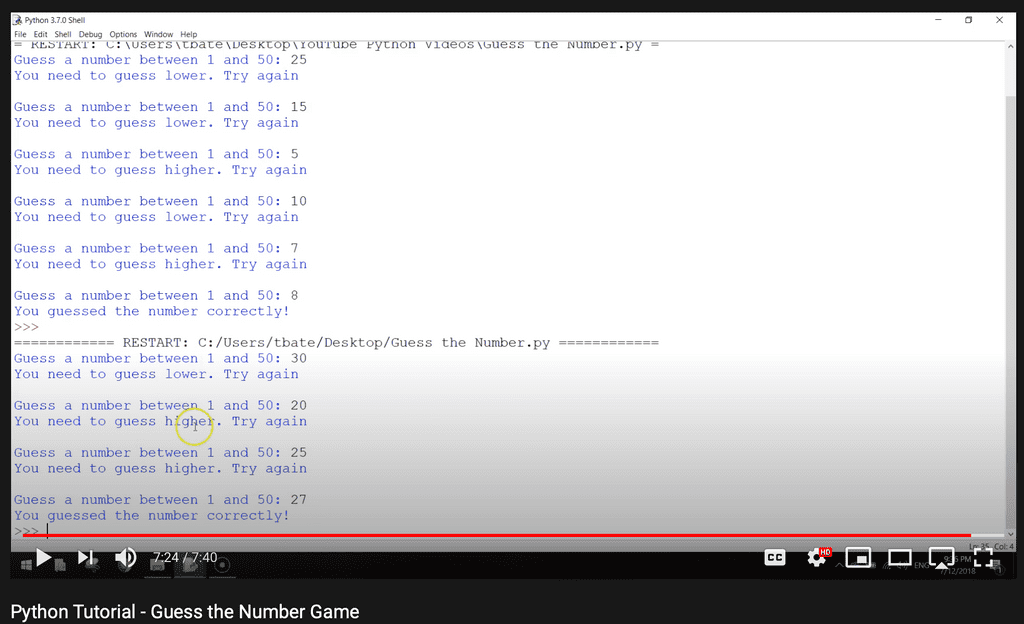
Is a palindrome
Ask the user to give you five words. Then check if each of the five words is a palindrome.
A palindrome is a word that remains the same whether it's read forward or backward.
Example:
madam
is a palindrome.- so is
malayalam
. - But not
geeks
.
Here's a youtube tutorial on coding up a palindrome checker in JavaScript.
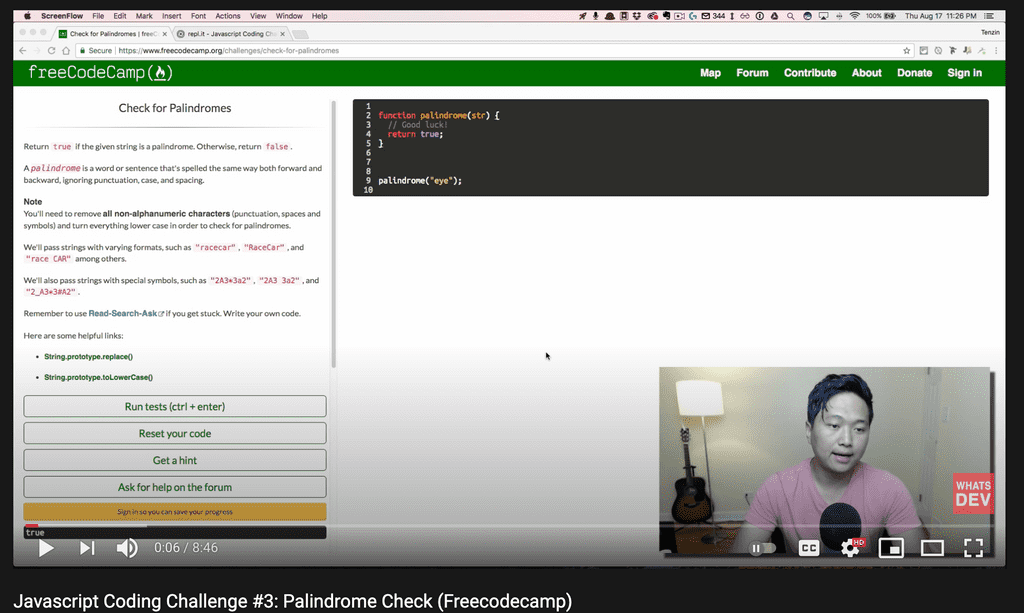
Email slicer
Collect an email address from the user and then find out if the user has a custom domain name or a popular domain name. For example:
-
Input:
mary.jane@gmail.com
- Output:
Hey Mary, I see your email is registered with Google. That's cool!
.
- Output:
-
Input:
peter.pan@myfantasy.com
- Output:
Hey Peter, looks like you've got your own custom setup at MyFantasy. Impressive!
.
- Output:
This is one of the convenient python projects that has a lot of use in the future. The program helps get you the username and domain name from an email address. You can even customize the application and send a message to the host with this information.
Lyrics generator
Ask a user to choose from a list of 10 songs. When the user does, you print out the lyrics to the song they selected.
Example:
Welcome, please select a select a song from this top 10 songs:
1. Baby by Bieber
2. Hotline Bling by Drake
3. Flawless by Beyonce
4. Fall by Eminem...
You chose Flawless by Beyonce. Here you go:
------- Flawless by Beyonce ------------
I'm out that H, town coming coming down
I'm coming down, drippin' candy on the ground
H, Town, Town, I'm coming down, coming down
Drippin' candy on the ground...
Press * to choose again.
Part 2 - Programming projects for beginner+
Use these coding projects to boost your skills beyond beginner levels.
If you’re completely new to the world of programming, this section is not for you. Instead, follow the beginner section above and use these tips on where to start as an absolute beginner to programming.
Python
- 42 Exciting Python Project Ideas & Topics for Beginners [2020]
- 13 Project Ideas for Intermediate Python Developers
- Youtube - Learn How to Build 12 Python Projects in a 3-Hour Course
- Youtube - 5 Python Projects for Beginners ~ Tech With Tim
JavaScript
- 100+ JavaScript Projects for Beginners!
- 48 Amazing JavaScript Open Source for the Past Year (v.2019)
- 20+ Projects you can do with JavaScript
- Youtube - 3 Javascript Projects in less than 1 hour! HTML, CSS and Javascript! ~ Danny Thompson
- Youtube - Web Development Project Ideas for Beginners | Building beginner projects in Javascript
- Youtube - 5 Javascript Projects to Build (For Beginners) by Andrew Sterkowitz
- Youtube - 10 JavaScript Projects in 10 Hours - Coding Challenge
Java
- 170+ Java Project Ideas – Your entry pass into the world of Java
- 100+ Free Java mini projects with Source Code
- Java Project Ideas – 37 Exciting Java projects for Beginners
- 21 Java Projects Ideas for beginners
- 10 Free Java Projects for Beginners to Know in 2020
Mobile app development (Android/iOS)
- Top 230 Android Projects – Beginner & Advanced Project Ideas
- 110 Mobile App Ideas For 2021 That Haven’t Been Made Yet
- 49+ Mobile App Ideas for 2020 (That Haven't Been Made)
For aspiring game developers
- 49 Ideas for Game Clones to Code
- 20 Interesting game development projects for beginners
- Top 5 BEST games to code as a beginner
- Learn Python by Building 5 Games
Programming overall
- 100+ Projects covering games, Cyber Security, Networking, Machine Learning
- Florin Pop's project ideas to improve your coding skills
- Florin Pop's endless app ideas
- Computer Science Projects from GeeksForGeeks
- Florin Pop's 100 days - 100 projects challenge
- 42 Projects to Practice Programming Skills
- 40 Side Project Ideas for Software Engineers
Closing out
Working on these projects, you might hit a road block and get completely discouraged. When that happens, turn to these stories of developers from all kinds of backgrounds who made it to motivate yourself to keep going.
As you progress in your programming career, you'll occasionally stumble on periods where you feel completely lost.
That's okay – use this guide for beginners and junior developers and why do you want to be a developer to put you back on track.
Software development is a challenging field.
But if so many others have done, you too can do it.
Thanks for reading
- FREE Cheatsheet: I help new programmers and junior devs focus on what matters instead of endless trial and error. If you're interested in boosting your confidence and skills, checkout our free cheatsheet.
- Follow on Twitter: Have a question, I'm most active on Twitter – feel free to reach out to me. My DM is open.
Heads Up - I love research so I tend to back my advice and approach with concepts from Behavioral Psychology and Neuroscience.